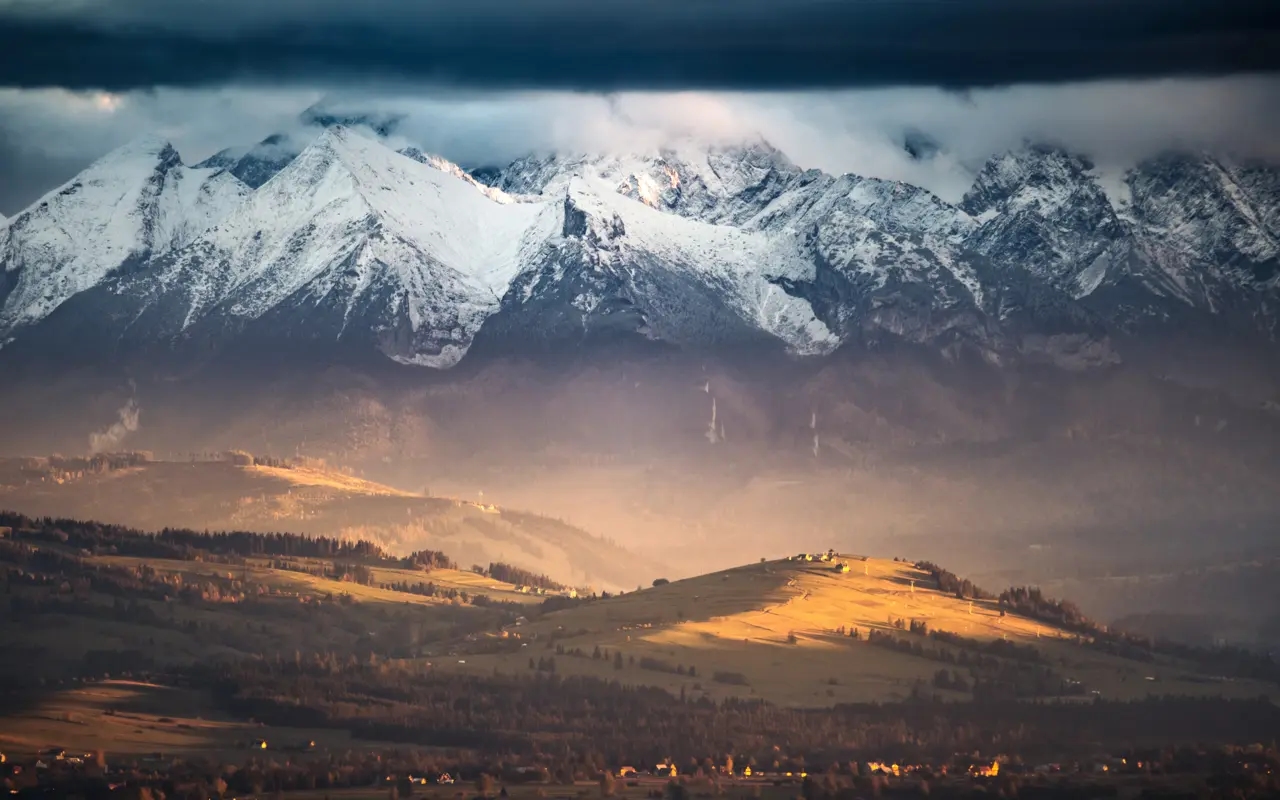
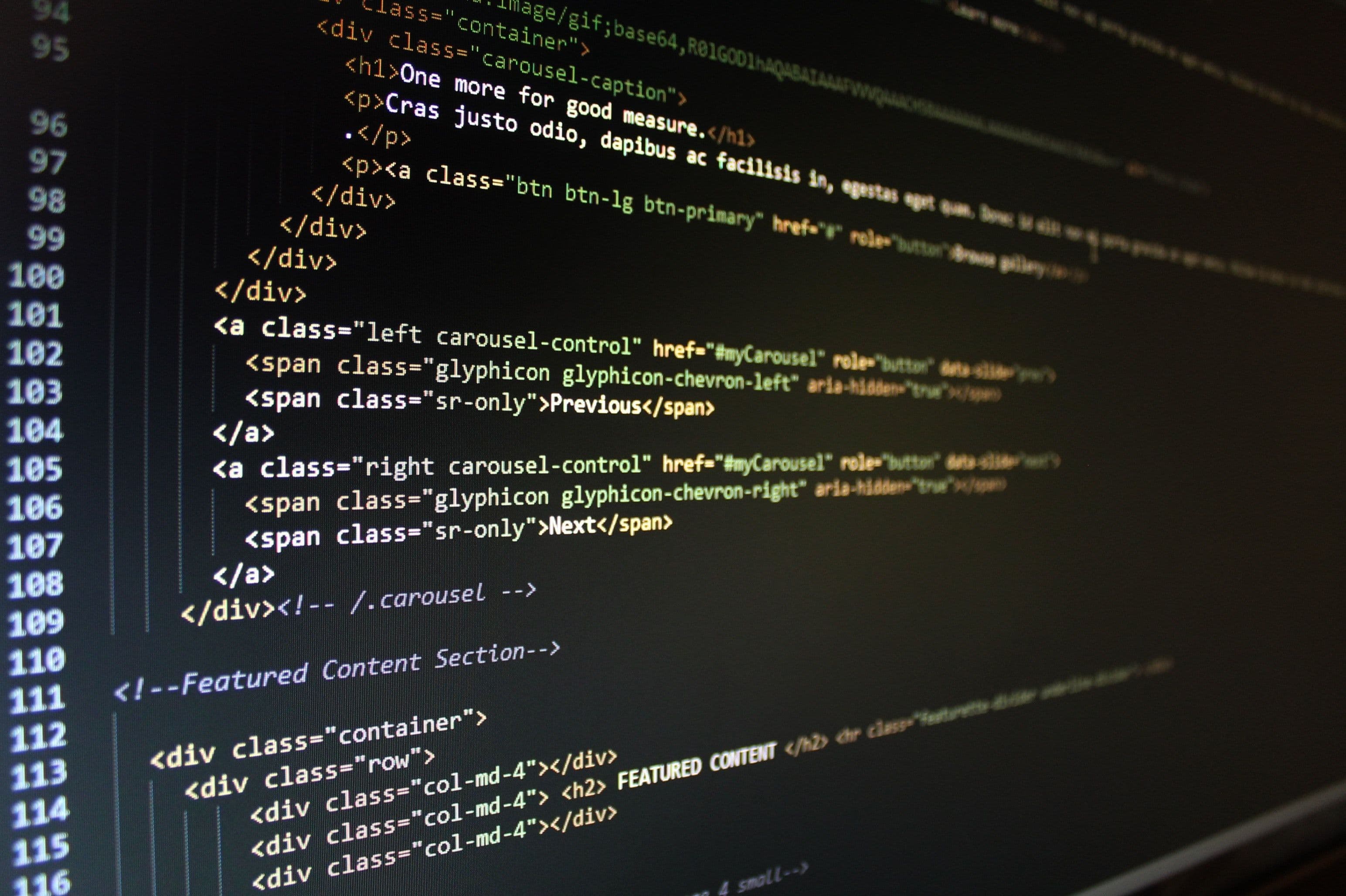
Introduction
Embarking on the journey of building a personal website can be both exciting and daunting. One must sift through the myriad of technologies available today, each promising to revolutionize the web development process. However, my recent project - building a high-performance website at zero cost - proved not only to be an insightful adventure into the modern tech stack, but also an intriguing exploration of AI's role in web development.
Choosing the Right Tools
One of the initial and most crucial steps was selecting the right technologies. After much consideration, my choices were Next.js, TypeScript, Tailwind CSS, and Sanity.io. Each of these tools offered unique advantages and, when combined, provided a powerful, flexible, and cost-effective solution for building and deploying a website.
Next.js
What sets Next.js apart is its ability to support both front-end and server-side operations in a single project, effectively acting as a full-stack framework. This feature is particularly showcased by the built-in API routes functionality, which allows you to write server-side code directly in your Next.js project.
For example, consider a scenario where we need to implement a simple email client. We can achieve this by creating an API route in Next.js. Here's how it might look in TypeScript:
// Using app router:
// app/api/contact/route.ts
export async function POST(req: Request) {
// Here you can use any email sending library you want.
// For simplicity, we'll just return a success status without actually sending an email.
const body: {
to: string;
message: string;
subject: string;
} = await req.json();
if (!to || !message || !subject) {
return res.status(400).json({ error: 'Missing required fields' });
}
// Here you would send the email and handle any errors.
res.status(200).json({ status: 'Email sent' });
}
This function could be used to send an email from your application. Being serverless, it's executed on demand and you're only charged for the compute time you consume - making it highly cost-effective.
What's more, Next.js's tight integration with Vercel means that deploying this full-stack application (both your front-end and your server-side email client) is an effortless process. This ease of use, combined with its zero-cost deployment, makes Next.js an ideal choice for projects of any scale.
TypeScript
At the core of modern web development, TypeScript stands as a game-changing technology. TypeScript, a statically-typed superset of JavaScript, brings an enhanced level of robustness and predictability to your codebase.
One significant advantage of TypeScript is its static type-checking. It allows developers to be aware of potential bugs and discrepancies at compile-time rather than run-time. Let's look at an example to illustrate the power of TypeScript's type system:
// Define a type for our user
type User = {
name: string;
age: number;
};
// This function expects a User object
function greet(user: User) {
return `Hello, ${user.name}! You are ${user.age} years old.`;
}
// TypeScript will show an error at compile-time because we are passing a number instead of a User object
greet(27);
In this example, TypeScript's type checking prevents us from passing a number to the greet function, which expects a User object. This early error detection can save developers from potential bugs and hours of debugging.
In addition, TypeScript supports advanced types, like intersection types, union types, and literal types, enabling developers to write more expressive and safer code.
Lastly, using TypeScript with Next.js is a breeze. Next.js provides built-in TypeScript support — just install the TypeScript package, add a tsconfig.json file, and you're ready to go. TypeScript files can be used for both front-end components and API routes, making it a full-stack language in a Next.js project.
All these aspects combined make TypeScript an invaluable tool in the modern web development toolbox.
Tailwind CSS
Tailwind CSS breaks away from traditional CSS frameworks by adopting a utility-first approach. Instead of pre-defined components, it provides low-level utility classes that let you build completely custom designs without ever leaving your HTML.
One of the biggest benefits of using Tailwind CSS is the speed at which you can build a user interface. For example, creating a responsive card component with a blue title is as simple as this:
<div class="max-w-md mx-auto bg-white rounded-xl shadow-md overflow-hidden md:max-w-2xl m-4">
<div class="md:flex">
<div class="md:flex-shrink-0">
<img class="h-48 w-full object-cover md:h-full md:w-48" src="/img/store.jpg" alt="Man looking at item at a store">
</div>
<div class="p-8">
<div class="uppercase tracking-wide text-sm text-indigo-500 font-semibold">Case study</div>
<a href="#" class="block mt-1 text-lg leading-tight font-medium text-black hover:underline">Finding customers for your new business</a>
<p class="mt-2 text-gray-500">Getting a new business off the ground is a lot of hard work. Here are five ideas you can use to find your first customers.</p>
</div>
</div>
</div>
This HTML snippet uses various utility classes provided by Tailwind CSS to style the card component. All the styling is done directly in the HTML markup, which makes it easy to see what styles are applied to each element.
Moreover, Tailwind CSS is configured via a tailwind.config.js file, making it incredibly customizable. You can define your color palette, typography scale, breakpoints, and more to match your design requirements.
Another important feature of Tailwind CSS is its responsive design capabilities. By simply prefixing a utility with sm:, md:, lg:, or xl:, you can apply styles at different screen sizes. This makes building responsive layouts straightforward and intuitive.
Lastly, Tailwind CSS plays well with Next.js and TypeScript. You can create React components using Tailwind CSS classes in your JSX, and these components can be strongly typed with TypeScript. This combination allows for rapid development of beautiful, type-safe components.
Tailwind CSS with Next.js's Server-Side Rendering (SSR)
One of the standout features of Next.js is its built-in support for server-side rendering (SSR). SSR allows for the rendering of pages on the server before they reach the client. This process results in faster page loads, better SEO, and an improved overall user experience.
When using Tailwind CSS with Next.js, it's essential to ensure that the styles are correctly applied during the server-side rendering process. Thankfully, Next.js and Tailwind CSS work harmoniously together in this regard.
By default, Tailwind CSS generates a large amount of utility classes, many of which might not be used in a project. When combined with Next.js's SSR, Tailwind CSS's "PurgeCSS" feature becomes incredibly valuable. It strips out unused CSS, ensuring that only the necessary styles are sent to the client. This not only reduces the size of the CSS payload but also ensures that the rendered content matches the final appearance right from the start, preventing any flash of unstyled content.
Here's a basic configuration of how you'd set up PurgeCSS with Tailwind in a Next.js project (has been renamed to "content" in v3.0):
// tailwind.config.js
module.exports = {
content: ['./app/**/*.{js,ts,jsx,tsx}', './components/**/*.{js,ts,jsx,tsx}'],
darkMode: false,
theme: {
extend: {},
},
variants: {},
plugins: [],
}
Sanity.io
Sanity.io is a headless CMS designed to handle structured content in a versatile and efficient manner. It provides developers with the flexibility to define their data structure according to their unique requirements. This data can then be queried in real-time, providing instant updates to any changes made.
One of the key features of Sanity.io is its content structuring. Content types, or 'schemas', in Sanity.io are defined using simple JavaScript objects. For example, a blog post schema might look like this:
export default defineType({
name: 'author',
title: 'Author',
type: 'document',
fields: [
defineField({
name: 'name',
title: 'Name',
type: 'string',
}),
...
})
This schema defines a blog post with a title field. You can add as many fields as you need, such as a slug, main image, publication date, and author reference.
Sanity.io also includes a powerful querying language called GROQ (Graph-Relational Object Queries). This allows you to query your data with high precision and flexibility. For example, to fetch all published blog posts and their authors, you could use a GROQ query like this:
*[_type == "blogPost" && publishedAt < now()]{
title,
slug,
publishedAt,
"authorName": author->name
}
However, Sanity.io also provides GraphQL support out-of-the-box. This means that if you prefer using GraphQL, you can do so. Here's how the above query would look in GraphQL:
{
allPost(where: {slug: {current: {eq: "${slug}"}}}) {
title
slug {
current
}
publishedAt
mainImage {
asset {
url
}
}
leadRaw
bodyRaw
categories {
title
}
tags
author {
name
}
}
}
Sanity.io’s real-time content updates, flexible content structuring, and powerful querying capabilities make it a standout choice for managing and delivering structured content.
The Deployment
Vercel, a cloud platform for static sites and Serverless Functions, is built to deploy web projects including those made with Next.js. It offers a smooth and efficient way to get your Next.js application live, with features that complement the Next.js framework perfectly.
Here's a simple step-by-step guide on how to deploy a Next.js application on Vercel:
- 1. Push your code to a Git provider (like GitHub, GitLab, or Bitbucket). Vercel integrates directly with these providers.
- 2. Visit Vercel.com, sign up for a new account if you don't have one, and then click on the "Import Project" button.
- 3. Connect Vercel to your Git provider and choose the repository that contains your Next.js project.
- 4. Vercel automatically detects that your app is a Next.js project. You can configure build settings, but the default settings typically work for most Next.js applications.
- 5. Click "Deploy," and Vercel will do the rest. Your application will be built and deployed, and you'll receive a unique URL to access it.
Vercel also supports continuous deployment. Once you've set up your project, any changes you push to your Git repository will automatically trigger a new deployment. This seamless integration means you can focus on coding and leave the deployment process to Vercel.
Moreover, Vercel provides serverless functions, which are perfect for running backend code that complements your Next.js app. These functions can be written in TypeScript, providing a consistent full-stack TypeScript experience. For example, the email client we wrote with Next.js's API routes can be deployed as a serverless function on Vercel without any additional configuration.
With its focus on performance, developer experience, and zero-config deployments, Vercel is a top choice for deploying Next.js applications.
AI-Powered Web Development
In recent years, AI has been making significant strides in many fields, and web development is no exception. With the latest AI language models, like OpenAI's GPT-4, developers can now leverage AI to solve coding challenges, generate content, and even write blog posts.
GPT-4, an advanced version of its predecessor GPT-3, has several improvements that make it an invaluable tool for developers:
- Improved Performance: GPT-4 has a better understanding of context, can generate more relevant responses, and is capable of maintaining a conversation over a more extended series of exchanges.
- Understanding Code: One of the most exciting advancements in GPT-4 is its improved code interpretation abilities. It can understand code snippets, provide solutions to coding problems, and even suggest code improvements. This feature can be a boon during development, as you can ask the model to generate code snippets, debug issues, and more.
- Content Generation: GPT-4 excels at generating human-like text. It can be used to write content for websites, create blog posts, draft emails, and more. This capability can save significant time and effort in content generation tasks.
However, it's essential to be aware of the constraints of AI-powered development:
- Limited Understanding: While GPT-4 is powerful, it doesn't truly "understand" the content in the way humans do. It predicts the next piece of text based on the patterns it has learned during training. Therefore, it might not always provide the expected output, especially for complex or ambiguous queries.
- Dependence on Input: The quality of the output heavily depends on the quality of the input. If the input is unclear or lacks context, the output may not be as expected.
- Lack of Creativity: While GPT-4 can generate creative-looking text, it doesn't possess creativity in the human sense. It can't come up with novel concepts or ideas that it hasn't been trained on.
Despite these limitations, AI-powered development, with tools like GPT-4, is a step forward in the evolution of web development. It's an exciting area with immense potential, and we're just starting to scratch the surface of what's possible.
Oh and this blog was written with Chat-GPT as well... :)
Tutorials
byAnton Čorňák
.Tags: